In this tutorial I will show you how to convert XML into associative array in php. I have found several methods and library to convert XML into array and following is a simple script to convert xml into array. I am using file_get_contents() php method to read xml file data into string.
Steps to Convert XML into Associative Array
Step 1: Following is a sample XML file books.xml
<?xml version='1.0'?> <books> <book name='Java'> <reference>book1</reference> </book> <book name='PHP'> <reference>book2</reference> </book> <book name='Android'> <reference>book3</reference> </book> <book name='Web'> <reference>book4</reference> </book> </books>
Step 2: Convert books.xml file into string
I have used file_get_contents() PHP method to read entire file into a string and store into $xmlfile variable.
$xmlfile = file_get_contents('PATH/books.xml');
Step 3: Convert string of XML into an Object
I have used simplexml_load_string() php method to convert string of XML into an object.
$xmlobj = simplexml_load_string($xmlfile);
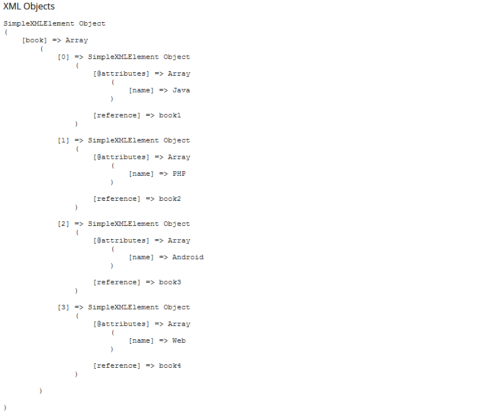
Step 4: Encode XML Object into JSON
To encode XML object into JSON use json_encode php method
$xmljson = json_encode($xmlobj);

Step 5: Decode the JSON Object
Now we will decode the json to get array from json string.
$booksData = json_decode($xmljson, true);
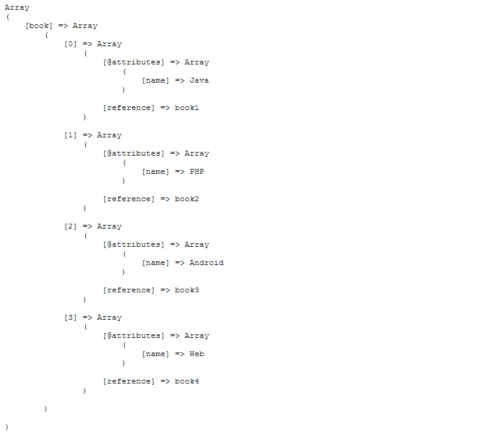
Following is the full Source Code to convert XML into associative array
$xmlfile = file_get_contents('PATH/books.xml'); $xmlobj = simplexml_load_string($xmlfile); $xmljson = json_encode($xmlobj); $booksData = json_decode($xmljson, true);