In this tutorial I will explain how you can Upload Multiple Files with Progress Bar. It will help you to show progress bar when you upload files.
Note PHP’s default max number of file upload is 20. You can not upload more than 20 files at once. To allow more than 20 files you need to edit php.ini file and change max_file_uploads value.
HTML Part: Following is the HTML of multiple files uploads.
<div class="container"> <div class="status"></div> <!-- multiple file upload form --> <form action="upload.php" method="post" enctype="multipart/form-data"> <input type="file" name="files[]" multiple="multiple" id="files"> <input type="submit" value="Upload" class="button"> </form> <!-- progress bar --> <div class="progress"> <div class="bar"> </div > <div class="percent">0%</div > </div> </div>
CSS Part: Now add following CSS to style this uploader:
.container { background: #fff; border: 1px solid #ccc; -webkit-border-radius: 5px; -moz-border-radius: 5px; border-radius: 5px; margin: 10px auto; padding: 10px 0; text-align: center; width: 600px; } .status { background: #008000; color: #fff; display: none; margin: 8px 0; padding: 5px; } .progress { margin: 10px auto; position: relative; width: 90%; } .bar { background: #008DE6; height: 20px; -webkit-border-radius: 5px; -moz-border-radius: 5px; border-radius: 5px; transition: width 0.3s ease 0s; width: 0; } .percent { color: #333; left: 48%; position: absolute; top: 0; } .button{ margin: 5px 0; }
Scripts: Now get jQuery form js and add as below:
<script type="text/javascript" src="js/jquery.js"></script> <script type="text/javascript" src="js/jquery.form.js"></script>
Progress Bar script: Following is the script to call form post and progress bar function.
$(function() { var status = $('.status'); var percent = $('.percent'); var bar = $('.bar'); $('form').ajaxForm({ dataType:'json', beforeSend: function() { status.fadeOut(); bar.width('0%'); percent.html('0%'); }, /* progress bar call back*/ uploadProgress: function(event, position, total, percentComplete) { var pVel = percentComplete + '%'; bar.width(pVel); percent.html(pVel); }, /* complete call back */ complete: function(data) { data = $.parseJSON(data.responseText); status.html(data.count + ' Files uploaded!').fadeIn(); } }); });
Save Files: Now create a file upload.php to save image files and return a JSON of number of files uploaded.
$max_size = 1024*200; $extensions = array('jpeg', 'jpg', 'png'); $dir = 'uploads/'; $count = 0; if ($_SERVER['REQUEST_METHOD'] == 'POST' and isset($_FILES['files'])) { foreach ( $_FILES['files']['name'] as $i => $name ) { if ( !is_uploaded_file($_FILES['files']['tmp_name'][$i]) ) continue; if ( $_FILES['files']['size'][$i] >= $max_size ) continue; if( !in_array(pathinfo($name, PATHINFO_EXTENSION), $extensions) ) continue; if( move_uploaded_file($_FILES["files"]["tmp_name"][$i], $dir . $name) ) $count++; } echo json_encode(array('count' => $count)); }
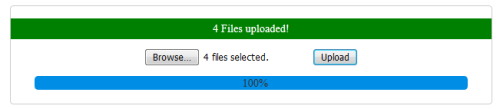
This is an example of upload multiple files with progress bar. Hope this will help you.