In this tutorial I explained how to customize list view with your custom style instead of using default list view style. In my previous tutorial I have explained how to parse json from a url and in this post in this post I will show you how to display data on customized list view.
So first we will create a layout “list_item.xml” for an item to display the website ID, Name and URL.
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" android:background="@drawable/item_background" > <TextView android:id="@+id/wid" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="75dp" android:layout_marginTop="15dp" /> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginTop="15dp" android:textStyle="bold" android:text="@string/wid" /> <TextView android:id="@+id/name" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_below="@+id/wid" android:layout_marginLeft="75dp" android:layout_marginTop="15dp" /> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_below="@+id/wid" android:layout_marginTop="15dp" android:text="@string/name" android:textStyle="bold" /> <TextView android:id="@+id/url" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_below="@+id/name" android:layout_marginLeft="75dp" android:layout_marginTop="15dp" /> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_below="@+id/name" android:layout_marginTop="15dp" android:textStyle="bold" android:text="@string/url" /> </RelativeLayout>
Now we will style item shape and corner radius in drawable as below:
item_background.xml <?xml version="1.0" encoding="UTF-8"?> <shape xmlns:android="http://schemas.android.com/apk/res/android" android:shape="rectangle"> <solid android:color="#FFFFFF"/> <stroke android:width="1dp" android:color="#C1C0C0" /> <corners android:bottomRightRadius="7dp" android:bottomLeftRadius="7dp" android:topLeftRadius="7dp" android:topRightRadius="7dp"/> </shape>
Now we will create a layout “activity_list.xml” for list view activity.
activity_list.xml <?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" android:orientation="vertical"> <ListView android:id="@+id/listview" android:layout_width="fill_parent" android:layout_height="fill_parent" android:divider="@null" android:dividerHeight="5dp" android:visibility="visible" android:smoothScrollbar="true" /> </LinearLayout>
Now we will create a activity “ListviewActivity.java” to get the data and display on customize list view.
package com.tricks.readjsonfromurl; import java.io.IOException; import java.util.ArrayList; import java.util.HashMap; import org.apache.http.HttpResponse; import org.apache.http.client.ClientProtocolException; import org.apache.http.client.HttpClient; import org.apache.http.client.methods.HttpPost; import org.apache.http.impl.client.DefaultHttpClient; import org.apache.http.util.EntityUtils; import org.json.JSONArray; import org.json.JSONException; import org.json.JSONObject; import android.app.Activity; import android.os.Bundle; import android.os.StrictMode; import android.widget.ListAdapter; import android.widget.ListView; import android.widget.SimpleAdapter; public class ListviewActivity extends Activity { ListView listView; ArrayList<HashMap<String, String>> arrList; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_list); listView = (ListView) findViewById(R.id.listview); arrList = new ArrayList<HashMap<String, String>>(); String json_str = getJsonData(); try{ JSONArray jArray = new JSONArray(json_str); for (int i = 0; i < jArray.length(); i++) { JSONObject json = null; json = jArray.getJSONObject(i); HashMap<String, String> map1 = new HashMap<String, String>(); // adding each child node to HashMap key => value map1.put("id", json.getString("id")); map1.put("name", json.getString("name")); map1.put("url", json.getString("url")); // adding HashList to ArrayList arrList.add(map1); } } catch ( JSONException e) { e.printStackTrace(); } if(!arrList.isEmpty()){ ListAdapter adapter = new SimpleAdapter( this, arrList, R.layout.list_item, new String[] { "id", "name", "url" }, new int[] { R.id.wid, R.id.name, R.id.url }); listView.setAdapter(adapter); } } private String getJsonData(){ StrictMode.setThreadPolicy(new StrictMode.ThreadPolicy.Builder() .detectDiskReads() .detectDiskWrites() .detectNetwork() // or .detectAll() for all detectable problems .penaltyLog() .build()); String str = ""; HttpResponse response; HttpClient myClient = new DefaultHttpClient(); HttpPost myConnection = new HttpPost("http://demos.tricksofit.com/files/json2.php"); try { response = myClient.execute(myConnection); str = EntityUtils.toString(response.getEntity(), "UTF-8"); } catch (ClientProtocolException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } return str; } }
Finally in manifest file we will add ListviewActivity as main activity
<activity android:name="com.tricks.readjsonfromurl.ListviewActivity" android:label="@string/app_name" > <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity>
Now we will get the output as customize list view with data as below:
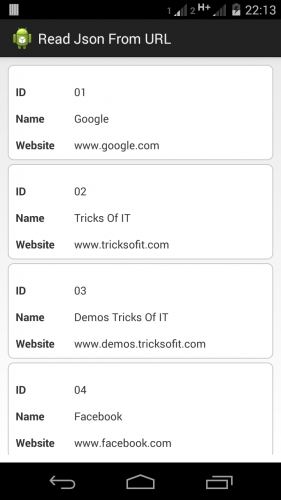